零基础小白入门必看篇:学习Python之面对对象基础( 二 )
print(wangcai.tooth) # 10 print(xiaohei.tooth) # 10 # 类属性的优点 # 类的实例记录的某项数据始终保持一致时,则定义类属性 # 实例属性要求每个对象为其单一开辟一份内存空间来记录数据, 而类属性为全类所共有 , 仅占用一份内存 , 更加节省内存空间 修改类属性类属性只能通过类对象修改 , 不能通过实例对象修改 , 如果通过实例对象修改类属性 , 表示的是创建了一个实例属性class Dog(object): tooth = 10 wangcai = Dog() xiaohei = Dog() # 修改类属性 Dog.tooth = 12 print(Dog.tooth) # 12 print(wangcai.tooth) # 12 print(xiaohei.tooth) # 12 # 不能通过对象修改属性, 如果这样操作,实则是创建了一个实例属性 wangcai.tooth = 20 print(Dog.tooth) # 12 print(wangcai.tooth) # 20 print(xiaohei.tooth) # 12
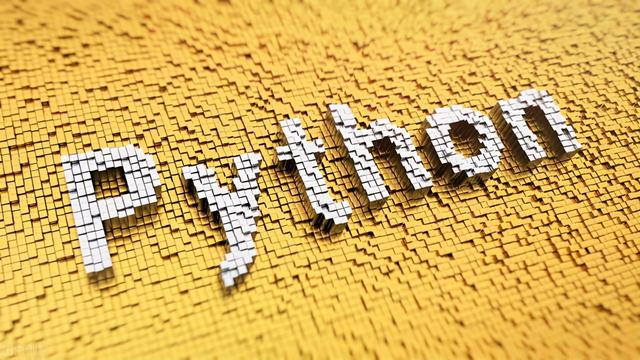
类方法和静态方法
- 类方法类方法的特点第一个形参是类对象的方法需要用装饰器@classmethod来标识其为类方法, 对于类方法 , 第一个参数必须是类对象, 一般以cls作为第一个参数类方法的使用场景当方法中需要使用类对象(如访问私有类属性等)时, 定义类方法类方法一般和类属性配合使用class Dog(object): __tooth = 10 @classmethod def get_tooth(cls): return cls.__tooth wangcai = Dog() result = wangcai.get_tooth() print(result) # 10
- 静态方法静态方法的特点需要通过装饰器@staticmethod来进行装饰 , 静态方法既不需要传递类对象也不需要传递实例对象(形参没有self/cls)静态方法也能够通过实例对象和类对象去访问静态方法使用场景当方法中即不需要使用实例对象(如实例对象,实例属性), 也不需要使用类对象(如类属性,类方法,创建实例等)时 , 定义静态方法取消不需要的参数传递,有利于减少不必要的内存占用和性能消耗class Dog(object): @staticmethod def info_print(): print('这是一个狗类, 用于创建狗实例') wangcai = Dog() # 静态方法既可以使用对象访问又可以使用类方法 wangcai.info_print() Dog.info_print()
- __ init __(): 初始化对象__init__()方法, 在创建对象时默认被调用,不需要手动调用__init__(self)中的self参数,不需要开发者传递 , python解释器会自动把当前的对象引用传递过去
class Washer():# 定义__init__ , 添加实例属性def __init__(self):# 添加实例属性self.width = 500self.height = 800def print_info(self):# 类里面调用实例属性print(f'洗衣机的宽度是{self.width},高度是{self.height}')haier1 = Washer()haier1.print_info()
- 带参数的__init__()
def print_info(self):print(f'洗衣机的宽度是{self.width}')print(f'洗衣机的高度是{self.height}')
haier1 = Washer(10, 20) haier1.print_info()haier2 = Washer(30, 40) haier2.print_info()
* \_\_str\_\_(): 当使用print()输出对象的时候,默认打印对象的内存地址 。 如果类定义了_\_str\_\_方法,那么就会打印从这个方法中return 的数据```pythonclass Washer():def __init__(self, width, height):self.width = widthself.height = heightdef __str__(self):return '这是海尔洗衣机的说明书'haier1 = Washer(10, 20)# 这是海尔洗衣机的说明书print(haier1)
推荐阅读
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-
- 苹果两款新iPad齐曝光:性能提高、入门款更轻薄、售价便宜
- RHEL 9提升了x86_64处理器的入门要求
- 视频小白们的外挂装备:百视悦R5监视器+T2提词器套装上手
- 市科委与联影集团联合首设“探索者计划”,共推基础及应用基础研究
- 入门HiFi享好声,这几款耳机绝对值得入手
- 从事Java开发时发现基础差,是否应该选择辞职自学一段时间
- 大力发展新型基础设施建设“数字浙江”再添新引擎
- 「新书推荐」5G安全:5G生态的重要组成部分和5G发展的关键基础条件
- DIY从入门到放弃:电源挑贵的买就靠谱吗?
- 微软Surface Pro 8基础版规格或升级 酷睿i3+8GB运存